Let's start with the coding of the level 1 game and more precisely with the main character. You need again to play the game in order to understand the pattern of the character's movement along with any interaction with the other characters or surroundings.
Here is the code for moving the main character:
if (key=VK_RIGHT) and (Blocked=false) then begin // Key Right
if PosChar=2 then begin PosChar:=3; ImageMan3.visible:=true; ImageMan2.visible:=false; PlaySound(pfPlay); end;
if PosChar=1 then begin PosChar:=2; ImageMan2.visible:=true; ImageMan1.visible:=false; PlaySound(pfPlay); end;
if PosChar=5 then begin PosChar:=6; ImageMan6.visible:=true; ImageMan5.visible:=false; PlaySound(pfPlay); end;
if PosChar=4 then begin PosChar:=5; ImageMan5.visible:=true; ImageMan4.visible:=false; PlaySound(pfPlay); end;
if PosChar=8 then begin PosChar:=9; ImageMan9.visible:=true; ImageMan8.visible:=false; PlaySound(pfPlay); end;
if PosChar=7 then begin PosChar:=8; ImageMan8.visible:=true; ImageMan7.visible:=false; PlaySound(pfPlay); end;
end;
if (key=VK_LEFT) and (Blocked=false) then begin // Key Left
if PosChar=2 then begin PosChar:=1; ImageMan1.visible:=true; ImageMan2.visible:=false; PlaySound(pfPlay); end;
if PosChar=3 then begin PosChar:=2; ImageMan2.visible:=true; ImageMan3.visible:=false; PlaySound(pfPlay); end;
if PosChar=5 then begin PosChar:=4; ImageMan4.visible:=true; ImageMan5.visible:=false; PlaySound(pfPlay); end;
if PosChar=6 then begin PosChar:=5; ImageMan5.visible:=true; ImageMan6.visible:=false; PlaySound(pfPlay); end;
if PosChar=8 then begin PosChar:=7; ImageMan7.visible:=true; ImageMan8.visible:=false; PlaySound(pfPlay); end;
if PosChar=9 then begin PosChar:=8; ImageMan8.visible:=true; ImageMan9.visible:=false; PlaySound(pfPlay); end;
end;
if (key=VK_DOWN) and (Blocked=false) then begin // Key Down
if PosChar=4 then begin PosChar:=1; ImageMan1.visible:=true; ImageMan4.visible:=false; end;
if PosChar=7 then begin PosChar:=4; ImageMan4.visible:=true; ImageMan7.visible:=false; end;
if PosChar=5 then begin PosChar:=2; ImageMan2.visible:=true; ImageMan5.visible:=false; end;
if PosChar=8 then begin PosChar:=5; ImageMan5.visible:=true; ImageMan8.visible:=false; end;
if PosChar=6 then begin PosChar:=3; ImageMan3.visible:=true; ImageMan6.visible:=false; end;
if PosChar=9 then begin PosChar:=6; ImageMan6.visible:=true; ImageMan9.visible:=false; end;
if PosChar=10 then begin PosChar:=9; ImageMan9.visible:=true; ImageMan10.visible:=false; end;
end;
if (key=VK_UP) and (Blocked=false) then begin // Key Up
if ((PosChar=10) and (BombCPos<>2)) then begin PosChar:=11; ImageMan11.visible:=true; ImageMan10.visible:=false; end;
if PosChar=9 then begin PosChar:=10; ImageMan10.visible:=true; ImageMan9.visible:=false; Score:=Score+20; end;
if PosChar=4 then begin PosChar:=7; ImageMan7.visible:=true; ImageMan4.visible:=false; Score:=Score+20; end;
if PosChar=1 then begin PosChar:=4; ImageMan4.visible:=true; ImageMan1.visible:=false; Score:=Score+20; end;
if PosChar=5 then begin PosChar:=8; ImageMan8.visible:=true; ImageMan5.visible:=false; Score:=Score+20; end;
if PosChar=2 then begin PosChar:=5; ImageMan5.visible:=true; ImageMan2.visible:=false; Score:=Score+20; end;
if PosChar=6 then begin PosChar:=9; ImageMan9.visible:=true; ImageMan6.visible:=false; Score:=Score+20; end;
if PosChar=3 then begin PosChar:=6; ImageMan6.visible:=true; ImageMan3.visible:=false; Score:=Score+20; end;
if PosChar=0 then begin PosChar:=1; ImageMan1.visible:=true; ImageMan0.visible:=false; Score:=Score+20; end;
end;
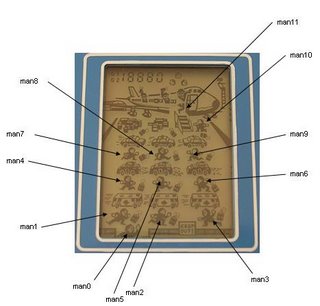